Algorithm To Convert Decimal To Binary
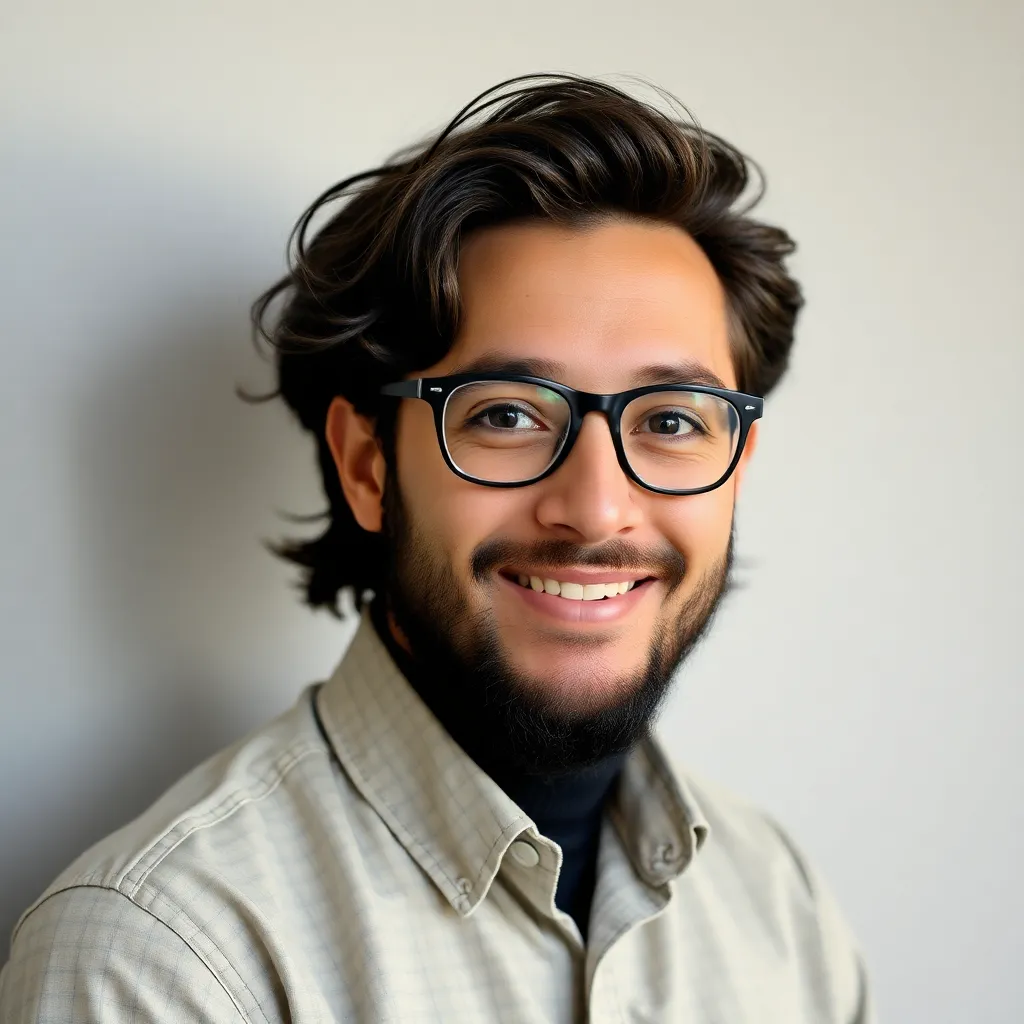
News Co
May 08, 2025 · 6 min read
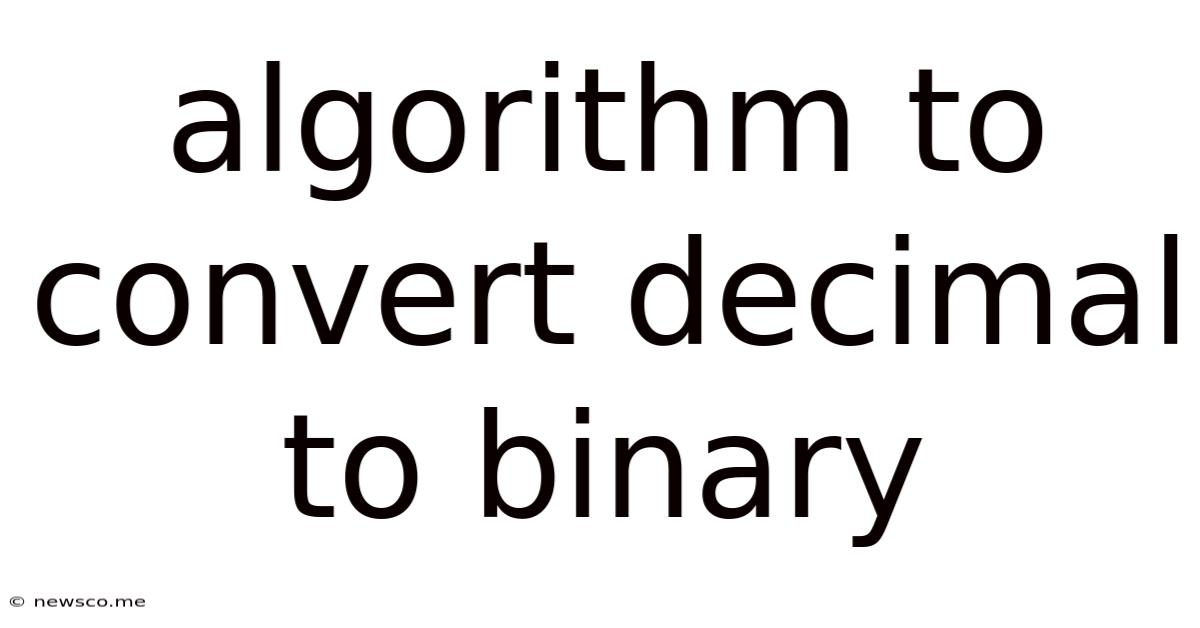
Table of Contents
Algorithm to Convert Decimal to Binary: A Comprehensive Guide
Converting decimal numbers to their binary equivalents is a fundamental concept in computer science and digital electronics. Understanding the underlying algorithms is crucial for anyone working with data representation, programming, or digital systems. This comprehensive guide will delve deep into various algorithms for decimal-to-binary conversion, explaining their workings, complexities, and applications. We'll cover everything from basic methods suitable for manual calculation to optimized algorithms for efficient computer processing.
Understanding the Decimal and Binary Number Systems
Before diving into the algorithms, let's briefly review the two number systems involved:
Decimal (Base-10): This is the number system we use daily. It uses ten digits (0-9) and each position represents a power of 10. For example, the number 1234 is interpreted as:
(1 × 10³) + (2 × 10²) + (3 × 10¹) + (4 × 10⁰)
Binary (Base-2): This system uses only two digits, 0 and 1. Each position represents a power of 2. For example, the binary number 1011 is:
(1 × 2³) + (0 × 2²) + (1 × 2¹) + (1 × 2⁰) = 8 + 0 + 2 + 1 = 11 (in decimal)
Algorithm 1: Repeated Division by 2 (The Most Common Method)
This is arguably the most straightforward and widely used algorithm for decimal-to-binary conversion. It involves repeatedly dividing the decimal number by 2 and recording the remainders. The remainders, read in reverse order, form the binary equivalent.
Steps:
- Divide: Divide the decimal number by 2.
- Record Remainder: Note the remainder (either 0 or 1).
- Repeat: Repeat steps 1 and 2 with the quotient obtained in step 1, until the quotient becomes 0.
- Reverse: Reverse the order of the remainders. This sequence of remainders is the binary representation of the original decimal number.
Example: Converting 13 (decimal) to binary:
Division | Quotient | Remainder |
---|---|---|
13 / 2 | 6 | 1 |
6 / 2 | 3 | 0 |
3 / 2 | 1 | 1 |
1 / 2 | 0 | 1 |
Reading the remainders in reverse order (1101), we get the binary equivalent of 13.
Code Implementation (Python):
def decimal_to_binary(decimal_num):
"""Converts a decimal number to its binary equivalent."""
if decimal_num == 0:
return "0" # Handle the special case of 0
binary_representation = ""
while decimal_num > 0:
remainder = decimal_num % 2
binary_representation = str(remainder) + binary_representation # Prepend remainder
decimal_num //= 2 # Integer division
return binary_representation
#Example usage
decimal_number = 25
binary_number = decimal_to_binary(decimal_number)
print(f"The binary representation of {decimal_number} is {binary_number}")
Algorithm 2: Subtraction Method
This method is less efficient than repeated division but provides a different approach to understanding the conversion process. It involves successively subtracting the largest possible power of 2 from the decimal number until it reduces to 0.
Steps:
- Find Largest Power: Determine the largest power of 2 that is less than or equal to the decimal number.
- Subtract: Subtract this power of 2 from the decimal number.
- Record: Record a '1' in the corresponding binary position (corresponding to the power of 2).
- Repeat: Repeat steps 1-3 with the remaining decimal value until the value becomes 0. Record a '0' for any power of 2 that wasn't subtracted.
Example: Converting 13 (decimal) to binary:
- 2³ = 8 ≤ 13. Subtract 8, leaving 5. Binary representation so far: 1___ (2³)
- 2² = 4 ≤ 5. Subtract 4, leaving 1. Binary representation: 11__ (2², 2³)
- 2¹ = 2 ≤ 1. Subtract 2, leaving -1. This is an error because we subtracted too much. Instead, try 2¹=2 >1. Record a 0 for 2¹
- 2⁰ = 1 ≤ 1. Subtract 1, leaving 0. Binary representation: 1101 (2³, 2², 2⁰)
Code Implementation (Python -Illustrative, not optimized):
def decimal_to_binary_subtraction(decimal_num):
"""Illustrative subtraction method (not optimized for efficiency)."""
if decimal_num == 0:
return "0"
binary = ""
power = 0
while 2**power <= decimal_num:
power += 1
power -= 1
while decimal_num > 0:
if 2**power <= decimal_num:
binary = "1" + binary
decimal_num -= 2**power
else:
binary = "0" + binary
power -= 1
return binary
This subtraction method demonstrates the principle but isn't as computationally efficient as the repeated division method. It’s important to note the error handling needed in step 3 to prevent incorrect results.
Algorithm 3: Using Built-in Functions (Python)
Python offers a built-in function bin()
that directly converts a decimal integer to its binary representation as a string. This is the most efficient method for practical applications.
Example:
decimal_number = 42
binary_string = bin(decimal_number)
print(f"The binary representation of {decimal_number} is {binary_string[2:]}") # [2:] removes the "0b" prefix
Algorithm 4: Bit Manipulation (Advanced)
For those familiar with bitwise operations, a more efficient method involves manipulating bits directly. This method is generally faster for large numbers because it avoids the overhead of repeated division or subtraction. This approach is commonly used in low-level programming or embedded systems.
This approach uses bitwise AND (&
), right shift (>>
), and other bitwise operators to extract bits one by one.
Example (Conceptual):
Let's say we have the decimal number 13.
- Check the least significant bit using a bitwise AND with 1: 13 & 1 = 1 (This is the least significant bit).
- Right-shift the number by 1 bit: 13 >> 1 = 6
- Repeat steps 1 and 2 until the number becomes 0.
This directly extracts the bits, but requires understanding bitwise operations.
Optimizations and Considerations
The choice of algorithm depends on the specific context. For most programming tasks, using the built-in bin()
function in Python (or its equivalent in other languages) is the most efficient and recommended approach. However, understanding the repeated division algorithm is crucial for grasping the fundamental principles behind decimal-to-binary conversion.
For very large numbers or resource-constrained environments, specialized algorithms or bit manipulation techniques might be necessary to optimize for speed and memory usage.
Applications of Decimal to Binary Conversion
The ability to convert between decimal and binary is essential in various fields:
- Computer Science: Representing numbers and data within computer systems.
- Digital Electronics: Designing and working with digital circuits and logic gates.
- Networking: Understanding IP addresses and network protocols.
- Data Compression: Some compression algorithms use binary representations for efficient data storage.
- Cryptography: Binary representations are fundamental to many encryption and decryption techniques.
Conclusion
Understanding decimal-to-binary conversion is fundamental to computer science and related fields. While several algorithms exist, the repeated division method provides a clear and intuitive approach for beginners. For practical applications, using built-in functions like bin()
in Python is the most efficient and recommended approach. The choice of algorithm often depends on the specific needs and constraints of the application. This guide has provided a thorough overview, enabling you to choose and implement the best method for your specific requirements. Remember that the core concept—representing numbers using powers of 2—is essential regardless of the algorithm used.
Latest Posts
Related Post
Thank you for visiting our website which covers about Algorithm To Convert Decimal To Binary . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.