How To Find Area With Coordinates
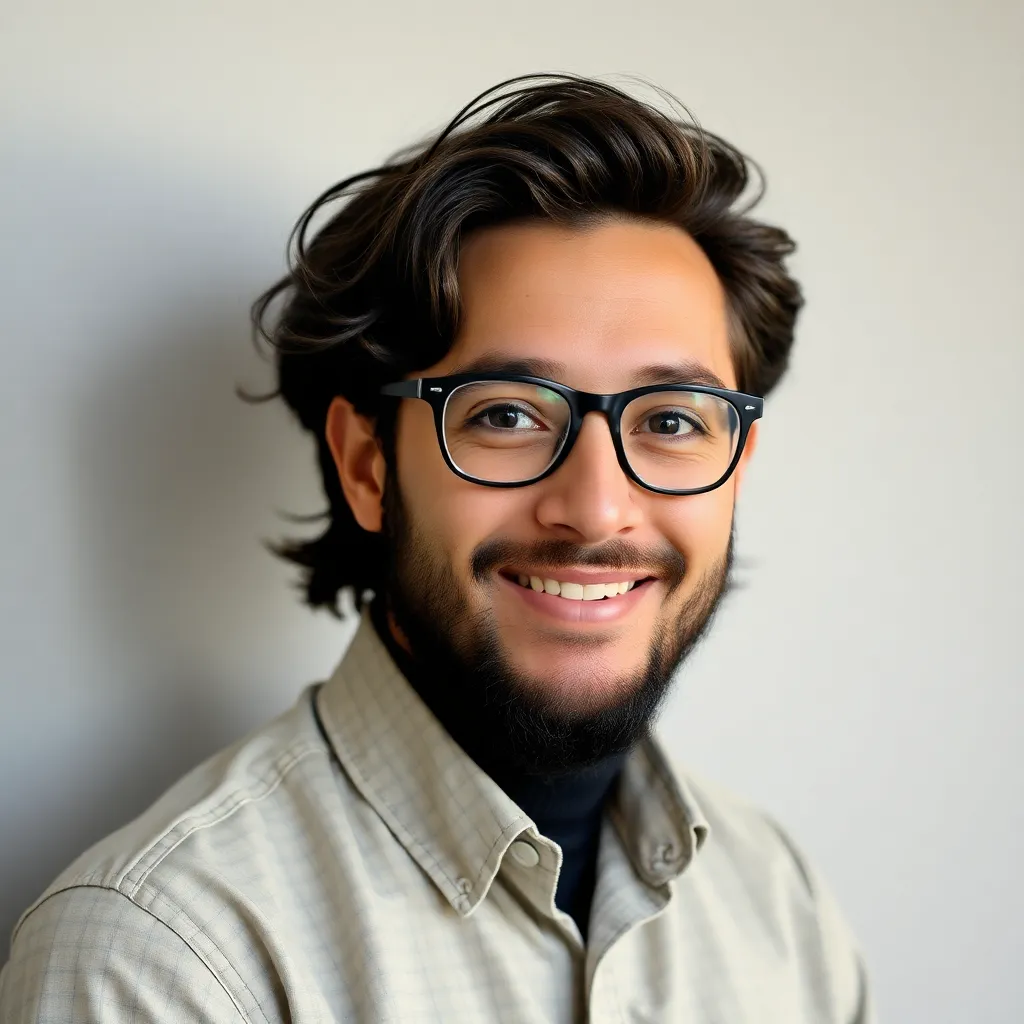
News Co
May 07, 2025 · 5 min read
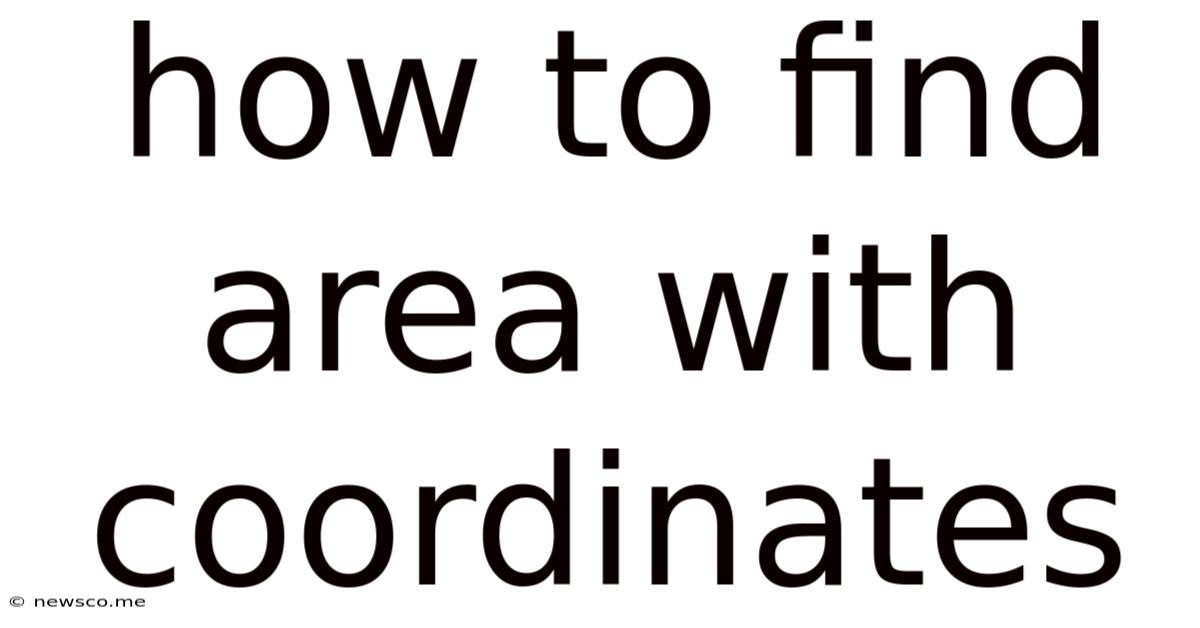
Table of Contents
How to Find Area with Coordinates: A Comprehensive Guide
Finding the area of a polygon given its coordinates is a common problem in various fields, from surveying and GIS to computer graphics and game development. While seemingly simple, the method depends on the type of polygon and the number of sides. This comprehensive guide will explore different techniques to calculate the area, ranging from simple triangles to complex polygons with numerous vertices. We'll also delve into the underlying mathematical principles and provide practical examples and code snippets to help you implement these methods.
Understanding the Basics: Area Calculation Principles
Before diving into specific methods, let's establish some fundamental concepts. The area of a polygon is essentially the two-dimensional space enclosed within its boundaries. Several formulas and algorithms can determine this area, depending on the shape's characteristics.
1. Triangles: The Building Block of Polygons
The most fundamental polygon is the triangle. Its area is calculated using the well-known formula:
Area = 0.5 * base * height
Where:
- base is the length of one side of the triangle.
- height is the perpendicular distance from the base to the opposite vertex.
However, if you only have the coordinates of the three vertices (let's say (x1, y1), (x2, y2), and (x3, y3)), you can use the determinant method:
Area = 0.5 * |x1(y2 - y3) + x2(y3 - y1) + x3(y1 - y2)|
This formula elegantly calculates the area directly from the coordinates without needing to explicitly find the base and height. The absolute value ensures a positive area.
2. Quadrilaterals: Beyond Triangles
Quadrilaterals, polygons with four sides, offer more complexity. The area calculation depends on the type of quadrilateral:
- Rectangle: Area = length * width
- Square: Area = side * side
- Parallelogram: Area = base * height (height is the perpendicular distance between parallel sides)
- Trapezoid: Area = 0.5 * (sum of parallel sides) * height
If you have the coordinates of the four vertices, the determinant method can be extended (though less elegant) or the quadrilateral can be divided into two triangles, calculating the area of each and summing them up.
Advanced Techniques for Complex Polygons
For polygons with more than four sides (pentagons, hexagons, etc.), the most efficient and versatile method is the Shoelace Formula (also known as the Surveyor's Formula). This formula works for any polygon, regardless of its shape or the order of vertices.
The Shoelace Formula: A Powerful Tool for Polygon Area Calculation
The Shoelace formula leverages the coordinates of the vertices to calculate the area:
Area = 0.5 * |(x1y2 + x2y3 + ... + xny1) - (y1x2 + y2x3 + ... + ynx1)|
Where:
- (xᵢ, yᵢ) are the coordinates of the i-th vertex.
- n is the number of vertices.
- The vertices should be listed in consecutive order (clockwise or counterclockwise).
Example: Let's say we have a polygon with vertices (0,0), (1,0), (1,1), (0,1). Using the Shoelace formula:
Area = 0.5 * |(00 + 11 + 11 + 00) - (01 + 01 + 10 + 10)| = 0.5 * |2 - 0| = 1
This correctly calculates the area of the unit square.
Implementing the Shoelace Formula in Different Programming Languages
The Shoelace formula is easily implemented in various programming languages. Here are examples in Python and JavaScript:
Python Implementation
def polygon_area(coordinates):
"""Calculates the area of a polygon given its coordinates using the Shoelace formula.
Args:
coordinates: A list of tuples, where each tuple represents a vertex (x, y).
Returns:
The area of the polygon.
"""
x = [coord[0] for coord in coordinates]
y = [coord[1] for coord in coordinates]
n = len(coordinates)
area = 0.5 * abs(sum(x[i] * y[(i + 1) % n] - x[(i + 1) % n] * y[i] for i in range(n)))
return area
#Example usage
coordinates = [(0,0), (1,0), (1,1), (0,1)]
area = polygon_area(coordinates)
print(f"The area of the polygon is: {area}")
coordinates = [(0,0), (1,1), (2,0)]
area = polygon_area(coordinates)
print(f"The area of the triangle is: {area}")
coordinates = [(1,1),(2,3),(4,1),(2,0)]
area = polygon_area(coordinates)
print(f"The area of the quadrilateral is: {area}")
JavaScript Implementation
function polygonArea(coordinates) {
let area = 0;
const n = coordinates.length;
for (let i = 0; i < n; i++) {
area += (coordinates[i][0] * coordinates[(i + 1) % n][1] -
coordinates[(i + 1) % n][0] * coordinates[i][1]);
}
return Math.abs(area) / 2;
}
// Example usage
const coordinates1 = [[0, 0], [1, 0], [1, 1], [0, 1]];
const area1 = polygonArea(coordinates1);
console.log(`The area of the polygon is: ${area1}`);
const coordinates2 = [[0,0], [1,1], [2,0]];
const area2 = polygonArea(coordinates2);
console.log(`The area of the triangle is: ${area2}`);
const coordinates3 = [[1,1],[2,3],[4,1],[2,0]];
const area3 = polygonArea(coordinates3);
console.log(`The area of the quadrilateral is: ${area3}`);
These code snippets provide a practical implementation of the Shoelace formula, allowing you to easily calculate the area of any polygon given its vertices' coordinates. Remember to handle potential errors like incorrect input formats.
Handling Self-Intersecting Polygons
The Shoelace formula, as presented above, assumes a simple polygon (no self-intersections). For self-intersecting polygons, the formula may produce incorrect results. More advanced algorithms, such as those based on triangulation or computational geometry techniques, are necessary to accurately calculate the area of such polygons. These algorithms are beyond the scope of this introductory guide but are readily available in computational geometry libraries.
Applications and Real-World Use Cases
The ability to calculate polygon areas from coordinates has numerous applications across diverse fields:
- Geographic Information Systems (GIS): Determining the area of land parcels, analyzing spatial data, and calculating distances.
- Computer Graphics: Rendering 2D shapes, calculating collision detection in games, and creating realistic simulations.
- Surveying and Mapping: Measuring land areas, creating accurate maps, and calculating volumes.
- Engineering and Construction: Calculating material quantities, designing structures, and optimizing layouts.
- Agriculture: Precision farming, monitoring crop yields, and managing resources efficiently.
Conclusion
Calculating the area of a polygon given its coordinates is a fundamental problem with many practical applications. While simple polygons can be handled using basic geometric formulas, the Shoelace formula provides a powerful and versatile solution for any polygon, regardless of its complexity (excluding self-intersecting polygons). Understanding the underlying mathematical principles and implementing the Shoelace formula in your preferred programming language empowers you to tackle a wide range of spatial problems efficiently and accurately. Remember to consider the limitations of the Shoelace formula when dealing with self-intersecting polygons and explore more advanced algorithms if necessary.
Latest Posts
Related Post
Thank you for visiting our website which covers about How To Find Area With Coordinates . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.