How To Multiply Vector Wise Equations
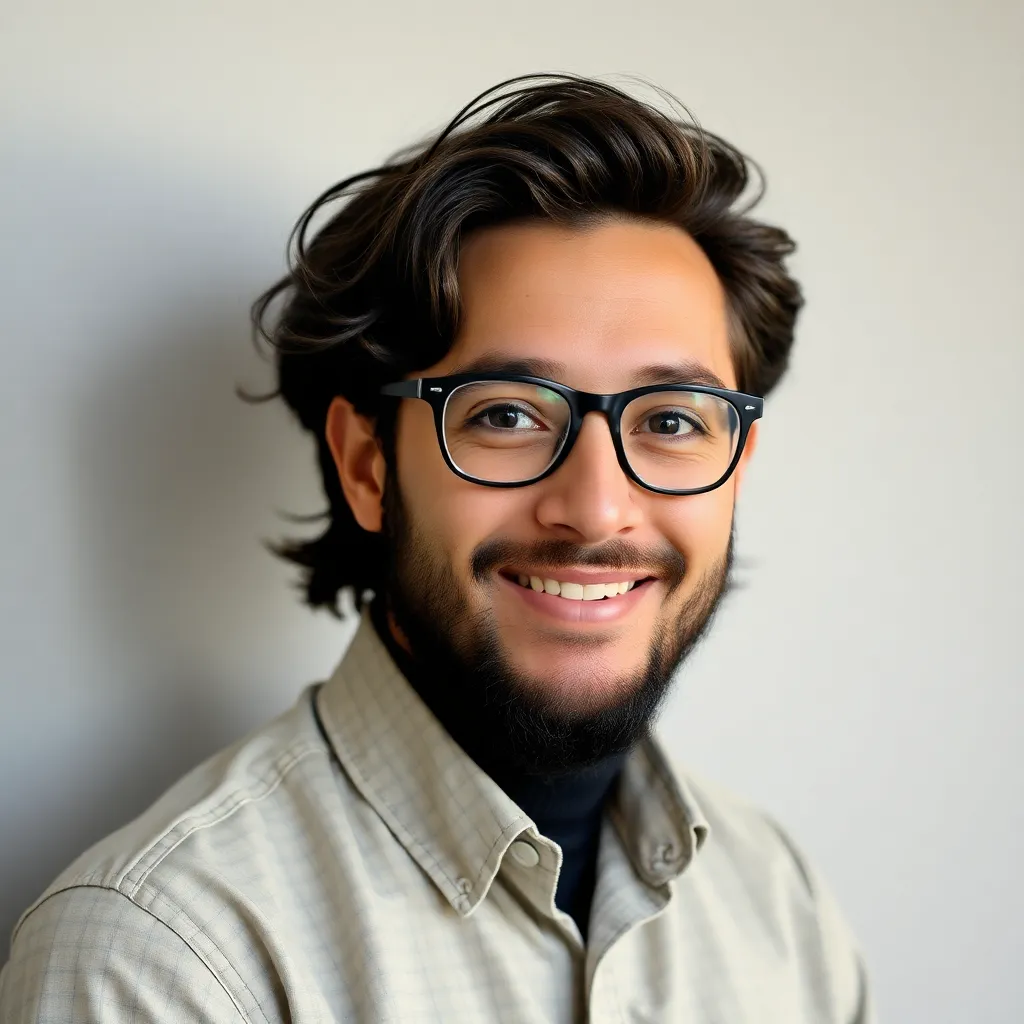
News Co
Mar 07, 2025 · 6 min read

Table of Contents
How to Multiply Vector-Wise Equations: A Comprehensive Guide
Vectorization is a powerful technique in linear algebra and programming that significantly speeds up computations by performing operations on entire arrays (vectors or matrices) simultaneously, rather than on individual elements. This is particularly crucial when dealing with large datasets, as it can dramatically reduce computation time. This article provides a comprehensive guide to multiplying vector-wise equations, covering various scenarios and techniques. We will explore different types of vector multiplication, their applications, and practical examples.
Understanding Vector Operations
Before delving into vector-wise multiplication, let's establish a foundational understanding of vectors and their operations. A vector is essentially an ordered collection of numbers. We can represent a vector as:
v = [v₁, v₂, v₃, ..., vₙ]
where v₁, v₂, v₃, ..., vₙ are the individual components or elements of the vector.
Several fundamental operations are defined for vectors, including:
- Addition: Adding two vectors involves adding their corresponding components: u + v = [u₁ + v₁, u₂ + v₂, u₃ + v₃, ..., uₙ + vₙ]
- Subtraction: Similar to addition, subtraction involves subtracting corresponding components: u - v = [u₁ - v₁, u₂ - v₂, u₃ - v₃, ..., uₙ - vₙ]
- Scalar Multiplication: Multiplying a vector by a scalar (a single number) involves multiplying each component by that scalar: cv = [cv₁, cv₂, cv₃, ..., cvₙ]
Types of Vector-Wise Multiplication
Vector-wise multiplication encompasses several distinct operations, each with its specific application and interpretation:
1. Element-wise Multiplication (Hadamard Product)
This is the most straightforward form of vector-wise multiplication. The Hadamard product, denoted by ⊙, multiplies corresponding components of two vectors of the same dimension.
u ⊙ v = [u₁v₁, u₂v₂, u₃v₃, ..., uₙvₙ]
Example:
Let u = [1, 2, 3] and v = [4, 5, 6]. Then:
u ⊙ v = [14, 25, 3*6] = [4, 10, 18]
Element-wise multiplication finds applications in various fields, including image processing (pixel-wise operations), machine learning (weight updates in neural networks), and signal processing.
2. Dot Product (Inner Product)
The dot product, denoted by u ⋅ v, is a scalar quantity obtained by summing the products of corresponding components of two vectors of the same dimension.
u ⋅ v = u₁v₁ + u₂v₂ + u₃v₃ + ... + uₙvₙ
Example:
Using the same vectors as above:
u ⋅ v = (14) + (25) + (3*6) = 4 + 10 + 18 = 32
The dot product has significant geometric interpretations, representing the projection of one vector onto another. It's crucial in calculating angles between vectors, projections, and work done by a force.
3. Cross Product (Vector Product)
The cross product is defined only for three-dimensional vectors. It results in a new vector that is orthogonal (perpendicular) to both input vectors. The cross product of u and v, denoted by u × v, is:
u × v = [(u₂v₃ - u₃v₂), (u₃v₁ - u₁v₃), (u₁v₂ - u₂v₁)]
Example:
Let u = [1, 2, 3] and v = [4, 5, 6]. Then:
u × v = [(26 - 35), (34 - 16), (15 - 24)] = [-3, 6, -3]
The cross product finds widespread use in physics, particularly in mechanics (torque calculation) and electromagnetism.
4. Matrix-Vector Multiplication
When dealing with matrices and vectors, matrix-vector multiplication becomes essential. A matrix is a rectangular array of numbers. Multiplying an m x n matrix A by an n x 1 vector v results in an m x 1 vector w:
w = A v
The element wᵢ is computed as the dot product of the i-th row of A and v.
Example:
Let A = [[1, 2], [3, 4]] and v = [5, 6]. Then:
w = [[1, 2], [3, 4]] [5, 6] = [(15 + 26), (35 + 46)] = [17, 39]
Practical Applications of Vector-Wise Multiplication
Vector-wise multiplication forms the bedrock of many computational algorithms across various disciplines. Here are some prominent examples:
-
Machine Learning: Neural networks extensively utilize vector-wise operations for weight updates during training, calculating activations, and performing forward and backward propagation. Element-wise multiplication is pivotal in applying activation functions.
-
Image Processing: Image manipulation tasks such as brightness/contrast adjustments, filtering, and edge detection heavily rely on element-wise operations on pixel matrices.
-
Computer Graphics: Vector-wise multiplications are crucial in rendering 3D scenes, transforming objects, applying lighting effects, and calculating intersections. Matrix transformations (rotations, scaling, translations) are fundamental.
-
Physics Simulations: Solving systems of linear equations, representing forces and velocities, and performing numerical integrations all involve matrix-vector multiplication and other vector operations.
-
Financial Modeling: Portfolio optimization, risk management, and forecasting often use matrix algebra and vector computations to analyze large datasets and model financial instruments.
-
Data Analysis: Vector-wise operations are instrumental in performing statistical computations, feature scaling, and data transformations in data analysis pipelines.
Coding Examples (Python with NumPy)
Python, coupled with the NumPy library, provides a powerful environment for performing vector-wise operations efficiently. NumPy's array operations are highly optimized for speed.
import numpy as np
# Element-wise multiplication
u = np.array([1, 2, 3])
v = np.array([4, 5, 6])
hadamard_product = u * v # NumPy automatically performs element-wise multiplication
print("Hadamard Product:", hadamard_product)
# Dot product
dot_product = np.dot(u, v) # Or u.dot(v)
print("Dot Product:", dot_product)
# Matrix-vector multiplication
A = np.array([[1, 2], [3, 4]])
w = np.dot(A, v)
print("Matrix-Vector Product:", w)
# 3D cross-product (requires 3D vectors)
u3d = np.array([1, 2, 3])
v3d = np.array([4, 5, 6])
cross_product = np.cross(u3d, v3d)
print("Cross Product:", cross_product)
This code demonstrates how concisely NumPy handles vector and matrix operations, avoiding explicit looping for improved efficiency. For very large datasets, consider libraries like CuPy which leverage GPUs for further speed improvements.
Advanced Techniques and Considerations
As you delve deeper into vector-wise computations, consider these advanced aspects:
-
Broadcasting: NumPy's broadcasting feature allows for operations between arrays of different shapes, provided certain conditions are met. This can simplify code and improve readability.
-
Vectorization vs. Looping: Always prioritize vectorized operations whenever possible. They are significantly faster than explicit looping in most cases.
-
Memory Management: For extremely large vectors and matrices, efficient memory management is crucial to prevent memory exhaustion. Techniques like memory mapping or using sparse matrix representations can be helpful.
-
Parallel Computing: Leverage parallel computing techniques (multiprocessing, multithreading) to accelerate computations further, especially when dealing with extensive datasets. Libraries like multiprocessing and concurrent.futures facilitate parallel processing in Python.
Conclusion
Vector-wise multiplication is a fundamental concept in linear algebra and numerical computing, offering significant advantages in terms of computational speed and code conciseness. Mastering these techniques is crucial for anyone working with large datasets, especially in fields like machine learning, image processing, and scientific computing. Through a thorough understanding of the various types of vector multiplication and their applications, along with utilizing efficient tools like NumPy, you can unlock the full potential of vectorized computations and significantly enhance the performance of your algorithms. Remember that efficient memory management and leveraging parallel computing techniques will further optimize your code for larger-scale computations. Continuous learning and experimentation with different vector operations will solidify your understanding and help you tackle complex computational problems effectively.
Latest Posts
Latest Posts
-
Find The Point On The Y Axis Which Is Equidistant From
May 09, 2025
-
Is 3 4 Bigger Than 7 8
May 09, 2025
-
Which Of These Is Not A Prime Number
May 09, 2025
-
What Is 30 Percent Off Of 80 Dollars
May 09, 2025
-
Are Alternate Exterior Angles Always Congruent
May 09, 2025
Related Post
Thank you for visiting our website which covers about How To Multiply Vector Wise Equations . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.