What Is A Point Of Concurrency
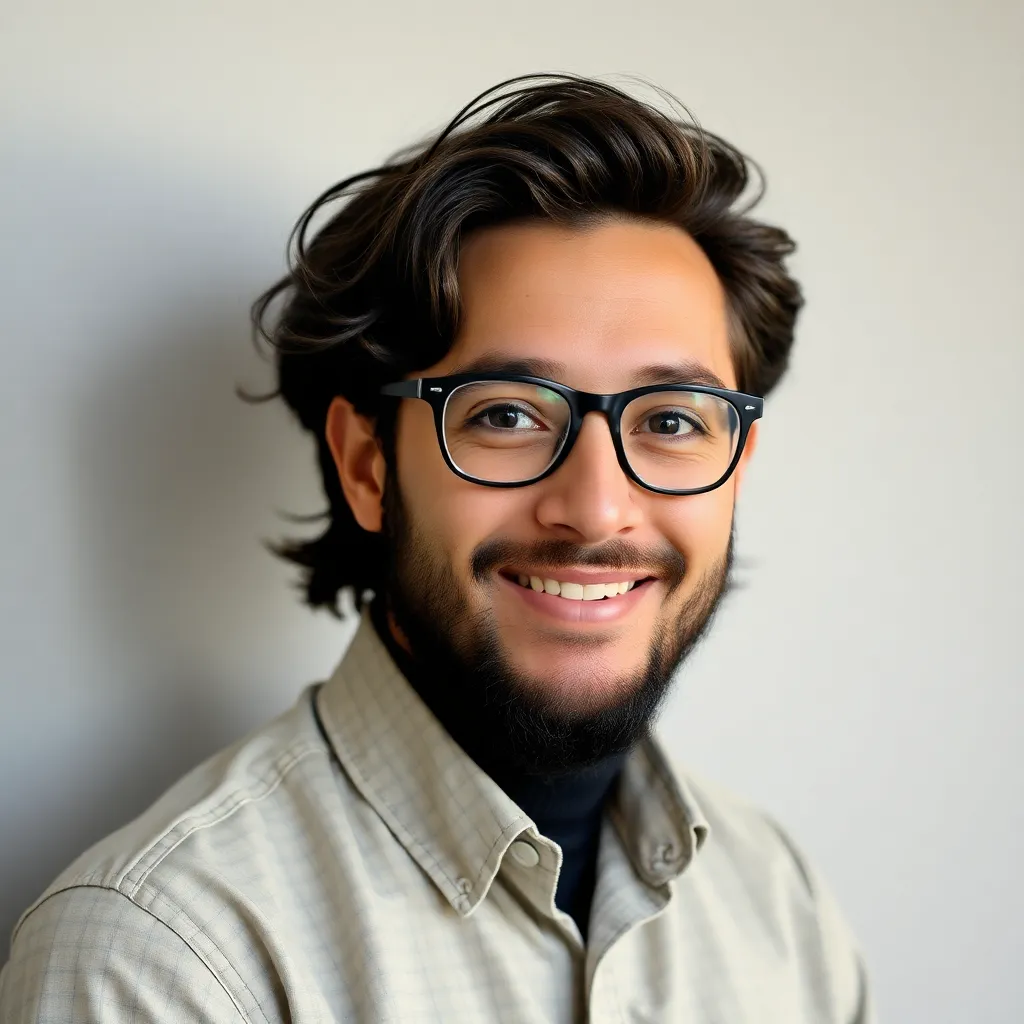
News Co
Apr 05, 2025 · 6 min read
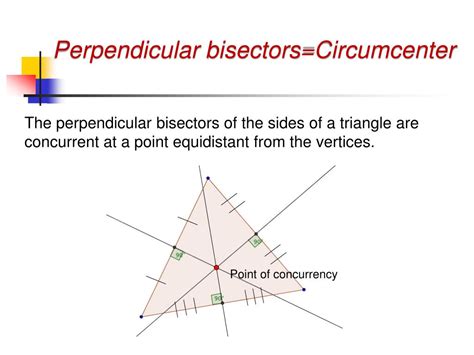
Table of Contents
What is a Point of Concurrency? Understanding Parallelism and Synchronization in Software Development
Concurrency, a cornerstone of modern software development, often sparks confusion. Understanding its core concept, the "point of concurrency," is crucial for writing efficient, reliable, and scalable applications. This article delves deep into the intricacies of concurrency, explaining what a point of concurrency is, its significance, and how to manage it effectively. We'll explore various concurrency models, common pitfalls, and best practices to help you master this vital aspect of programming.
Defining a Point of Concurrency: Where Threads Intersect
A point of concurrency, in its simplest form, is any place within a program where multiple threads of execution can potentially access or modify the same data simultaneously. This is also often referred to as a critical section. It represents a crucial juncture where the potential for race conditions—unpredictable behavior arising from multiple threads vying for the same resource—is high. Imagine a shared bank account: if multiple transactions attempt to withdraw money simultaneously without proper synchronization, the final balance could be incorrect. This is a classic example of a point of concurrency needing careful management.
The existence of a point of concurrency doesn't inherently imply a problem. The challenge arises when these points aren't handled correctly. Without proper mechanisms to control access, multiple threads could corrupt data, leading to unexpected results, program crashes, and data inconsistencies.
Identifying Points of Concurrency: A Practical Approach
Pinpointing points of concurrency in your code requires careful scrutiny. Look for shared resources accessed by multiple threads. This could include:
- Shared variables: Any variable accessible and modified by more than one thread.
- Global variables: By their very nature, these are prone to concurrent access issues.
- Static variables: Similar to global variables, these need careful management in concurrent environments.
- Files and I/O operations: When multiple threads read from or write to the same file simultaneously, data corruption can easily occur.
- Databases: Multiple threads interacting with a database require meticulous synchronization to avoid data inconsistencies.
- Network resources: Accessing network resources concurrently needs careful handling, particularly with shared sockets or connections.
Example (Illustrative Python):
Consider a simple counter incremented by multiple threads:
counter = 0 # Shared variable - a point of concurrency
def increment_counter():
global counter
for _ in range(100000):
counter += 1
# Multiple threads accessing and modifying 'counter' concurrently
Without proper synchronization, the final value of counter
will likely be less than 200000 (assuming two threads). This is because threads might be simultaneously reading and updating counter
, leading to lost updates. This simple example highlights the importance of recognizing and addressing points of concurrency.
Concurrency Models: Strategies for Managing Concurrent Access
Various models exist to handle points of concurrency effectively. Choosing the right model depends on the application's needs and complexity.
1. Mutexes (Mutual Exclusion): The Classic Approach
A mutex (mutual exclusion) is a synchronization primitive that ensures only one thread can access a shared resource at a time. It acts like a lock: a thread acquires the mutex before accessing the critical section and releases it afterward. This prevents other threads from entering the critical section while it's in use.
Example (Illustrative Python with threading
):
import threading
counter = 0
lock = threading.Lock() # Mutex
def increment_counter():
global counter
for _ in range(100000):
with lock: # Acquire and release the lock automatically
counter += 1
# ... (thread creation and execution) ...
The with lock:
block ensures that the critical section (counter += 1
) is protected. Only one thread can execute this block at a time.
2. Semaphores: Managing Resource Pools
A semaphore is a more general synchronization tool that allows a limited number of threads to access a shared resource concurrently. It maintains a counter representing the available resources. A thread acquires the semaphore by decrementing the counter (if the counter is > 0); otherwise, it blocks. When the thread finishes, it releases the semaphore by incrementing the counter. Mutexes are essentially semaphores with a counter of 1.
3. Condition Variables: Signaling and Waiting
Condition variables allow threads to wait for a specific condition to become true before continuing execution. They're often used in conjunction with mutexes. One thread might acquire a mutex, check a condition, and if it's false, wait on the condition variable. Another thread can signal the condition variable when the condition becomes true, waking up the waiting thread.
4. Monitors: High-Level Synchronization
Monitors encapsulate shared resources and the synchronization mechanisms needed to access them. They provide a higher-level abstraction, making concurrent programming easier and safer. They ensure mutual exclusion and often provide methods for waiting and signaling conditions.
Avoiding Common Pitfalls in Concurrent Programming
Several common pitfalls can lead to subtle and hard-to-detect bugs in concurrent programs:
- Deadlocks: A deadlock occurs when two or more threads are blocked indefinitely, waiting for each other to release resources. This is a classic concurrency problem.
- Race conditions: As mentioned earlier, race conditions occur when multiple threads access and modify shared data simultaneously, leading to unpredictable results.
- Starvation: A thread might be repeatedly denied access to a resource, effectively preventing it from making progress.
- Priority inversion: A high-priority thread might be blocked indefinitely because a lower-priority thread holds a resource it needs.
Best Practices for Concurrency Management
- Minimize shared resources: The fewer shared resources, the fewer points of concurrency and the lower the risk of concurrency problems.
- Use appropriate synchronization primitives: Choose the right synchronization mechanism (mutex, semaphore, condition variable, etc.) based on the specific needs of your application.
- Keep critical sections short: Minimize the time threads spend in critical sections to reduce the likelihood of conflicts and improve concurrency.
- Thorough testing: Concurrent programs are notoriously difficult to debug, so thorough testing is essential. Use tools that help you identify concurrency issues.
- Code reviews: Having another programmer review your concurrent code can help catch potential problems you might have missed.
- Consider thread pools: Thread pools can improve performance and resource management by reusing threads instead of constantly creating and destroying them.
Conclusion: Mastering the Art of Concurrency
Understanding points of concurrency is paramount for building robust and efficient concurrent applications. By carefully identifying these points, employing appropriate synchronization techniques, and adhering to best practices, you can effectively manage the challenges of concurrent programming. Remember that concurrency introduces complexity, but with careful planning and execution, you can harness its power to create highly scalable and responsive software. Consistent vigilance and a deep understanding of the underlying mechanisms are key to success in this domain. Continuous learning and adapting to new tools and methodologies are crucial for staying ahead in this ever-evolving field of software development.
Latest Posts
Latest Posts
-
What Is The Greatest Common Factor Of 60 And 45
Apr 05, 2025
-
Cuanto Son 180 Grados Celsius En Fahrenheit
Apr 05, 2025
-
Common Factors Of 28 And 36
Apr 05, 2025
-
How To Find The Perimeter Of An Isosceles Triangle
Apr 05, 2025
-
How Do You Convert A Fraction Into A Whole Number
Apr 05, 2025
Related Post
Thank you for visiting our website which covers about What Is A Point Of Concurrency . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.