Which Of The Following Is A Method Of Intersection
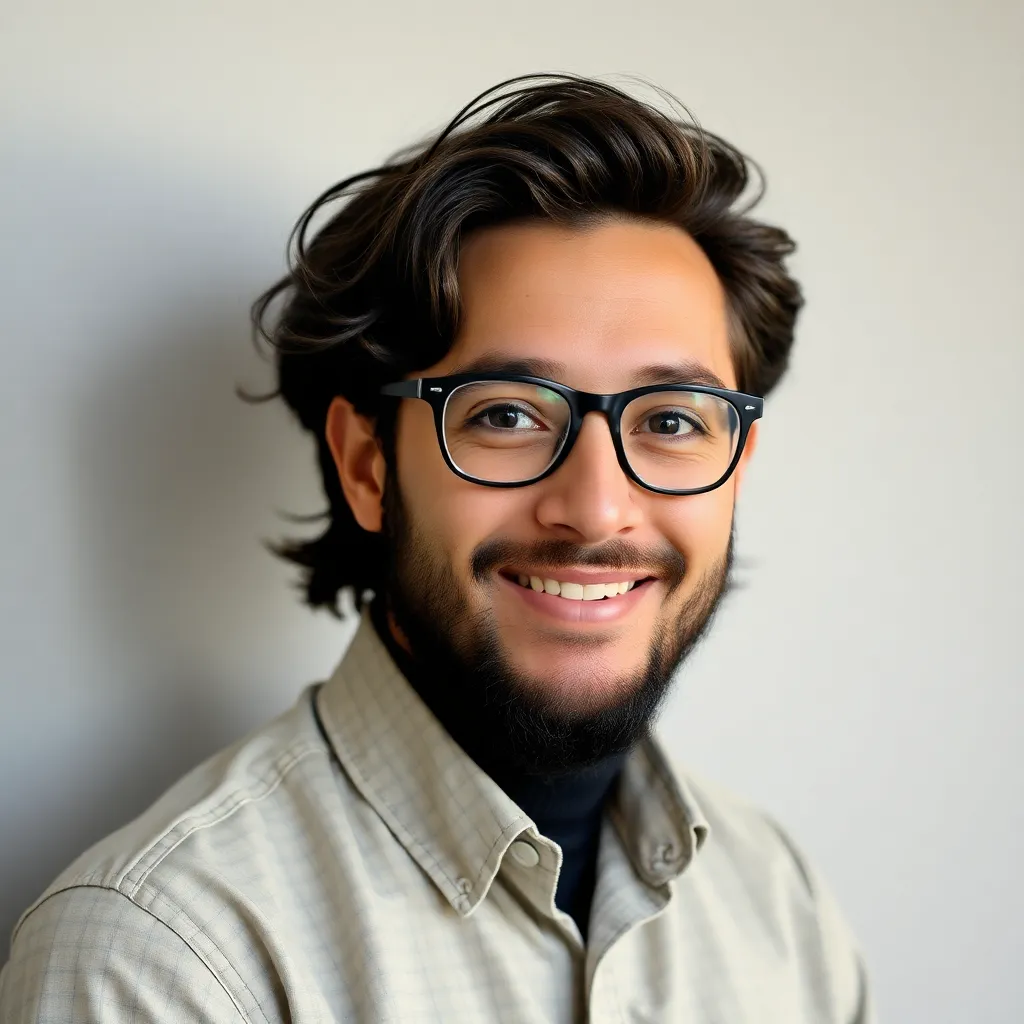
News Co
May 07, 2025 · 6 min read
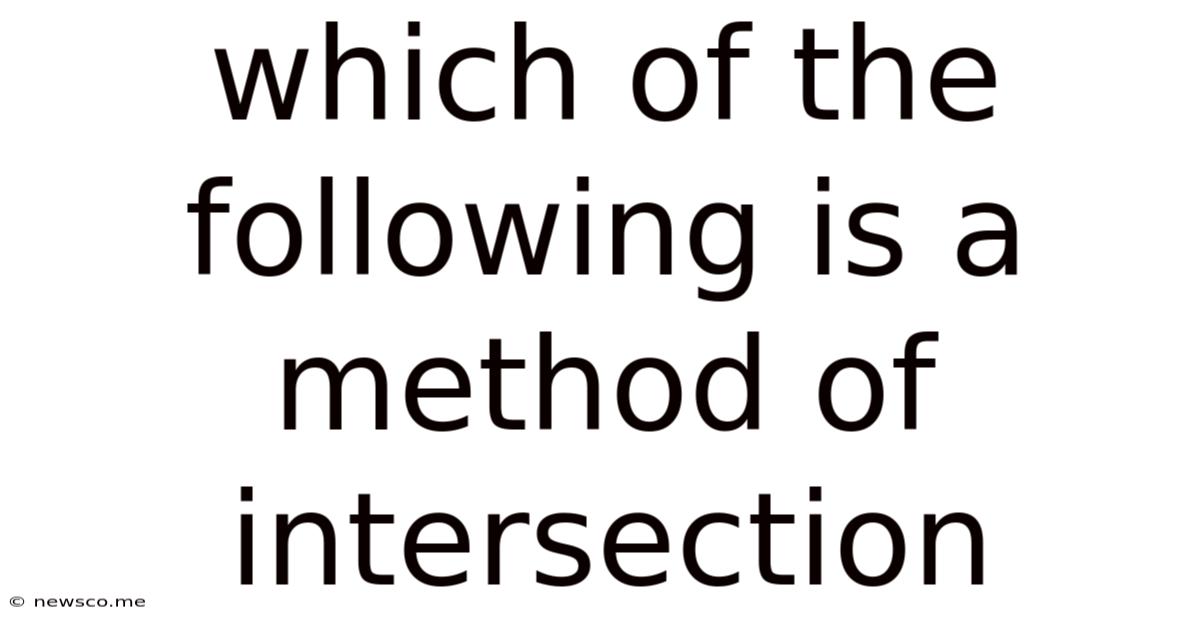
Table of Contents
Which of the Following is a Method of Intersection? Exploring Set Theory and Beyond
The question, "Which of the following is a method of intersection?" points to a fundamental concept in mathematics and computer science: set theory. Understanding intersection, and the various ways it's implemented, is crucial for anyone working with data structures, databases, or any field requiring the manipulation of collections of items. This article will delve deep into the methods of intersection, exploring its theoretical underpinnings, practical applications, and variations across different contexts.
Understanding Set Intersection: The Basics
In set theory, the intersection of two or more sets is a new set containing only the elements that are common to all the original sets. Imagine two circles overlapping; the intersection is the area where they both overlap. This concept is represented symbolically using the ∩ symbol. For example, if:
- Set A = {1, 2, 3, 4, 5}
- Set B = {3, 5, 6, 7, 8}
Then, the intersection of A and B (A ∩ B) is {3, 5}. Only 3 and 5 are present in both sets.
Key Properties of Set Intersection:
- Commutative: The order of the sets doesn't matter. A ∩ B = B ∩ A.
- Associative: When intersecting multiple sets, the grouping doesn't affect the result. (A ∩ B) ∩ C = A ∩ (B ∩ C).
- Idempotent: Intersecting a set with itself yields the same set. A ∩ A = A.
- Identity Element: The universal set (containing all elements) intersected with any set results in the original set. U ∩ A = A.
- Empty Set: The intersection of any set with the empty set (containing no elements) is always the empty set. A ∩ Ø = Ø.
Methods of Intersection: Algorithmic Approaches
The theoretical definition of intersection provides a solid foundation. However, implementing intersection practically requires algorithms. The efficiency and suitability of an algorithm depend on the size of the sets, the data structure used to represent them, and other constraints. Let's explore some common methods:
1. Brute-Force Method (Nested Loops):
This is the most straightforward approach, particularly suitable for smaller sets. It involves iterating through each element of the first set and checking if it exists in the second set.
Pseudocode:
function intersectionBruteForce(setA, setB):
intersectionSet = {}
for each element in setA:
if element exists in setB:
add element to intersectionSet
return intersectionSet
Time Complexity: O(n*m), where n and m are the sizes of setA and setB respectively. This is not efficient for large sets.
2. Using Hash Tables (Hash Sets):
Hash tables offer a significant performance improvement. Each element is hashed to a unique index, allowing for near-constant-time lookup. This dramatically speeds up the check for membership in the second set.
Pseudocode:
function intersectionHashTable(setA, setB):
hashSetB = create a hash set from setB
intersectionSet = {}
for each element in setA:
if hashSetB.contains(element):
add element to intersectionSet
return intersectionSet
Time Complexity: O(n + m) on average, where n and m are the sizes of setA and setB. This is much more efficient than the brute-force method, especially for large sets.
3. Sorted Arrays and Binary Search:
If the sets are represented as sorted arrays, a binary search can be employed to check for membership in the second set. This reduces the time complexity compared to a linear search within the nested loop approach.
Pseudocode:
function intersectionSortedArrays(sortedSetA, sortedSetB):
intersectionSet = {}
for each element in sortedSetA:
if binarySearch(sortedSetB, element):
add element to intersectionSet
return intersectionSet
Time Complexity: O(n log m), where n and m are the sizes of sortedSetA and sortedSetB respectively. This is more efficient than the brute-force method but less efficient than the hash table approach on average.
4. Merge-Based Intersection (for Sorted Sets):
If both sets are already sorted, a merge-like algorithm can efficiently compute the intersection. This algorithm iterates through both sets simultaneously, identifying common elements.
Pseudocode:
function intersectionMerge(sortedSetA, sortedSetB):
intersectionSet = {}
i = 0
j = 0
while i < length(sortedSetA) and j < length(sortedSetB):
if sortedSetA[i] == sortedSetB[j]:
add sortedSetA[i] to intersectionSet
i++
j++
else if sortedSetA[i] < sortedSetB[j]:
i++
else:
j++
return intersectionSet
Time Complexity: O(n + m), where n and m are the sizes of sortedSetA and sortedSetB. This is very efficient for sorted sets.
5. Set Operations in Databases (SQL):
Relational databases provide built-in set operations, including intersection. The INTERSECT
operator in SQL efficiently performs set intersection on the results of queries.
SQL Example:
SELECT column_name
FROM table1
INTERSECT
SELECT column_name
FROM table2;
Beyond Simple Sets: Intersection in Complex Data Structures
The concept of intersection extends beyond simple sets. We encounter it in various contexts:
1. Intersection of Lists:
Lists, unlike sets, allow duplicate elements. When intersecting lists, duplicates must be handled carefully. The result might contain multiple instances of the same element if it appears multiple times in both lists. Algorithms similar to those described above can be adapted to handle this.
2. Intersection of Arrays:
Arrays are similar to lists but are often implemented with a fixed size. Intersection algorithms for arrays are analogous to those for lists.
3. Intersection of Intervals:
Interval intersection is a common task in computational geometry and other fields. Given two intervals [a, b] and [c, d], their intersection is [max(a, c), min(b, d)] if the intersection is non-empty; otherwise, it's empty.
4. Intersection of Polygons:
Finding the intersection of two polygons involves more complex geometric algorithms. Libraries like Shapely (Python) provide functions for this.
5. Intersection in Graphs:
In graph theory, the intersection of two graphs can be defined in various ways, depending on the type of graph and the specific definition used. For example, the intersection of two graphs might be a new graph containing only the edges and nodes common to both original graphs.
Choosing the Right Method: Factors to Consider
The optimal method for finding the intersection depends on several factors:
-
Size of the Sets: For small sets, the brute-force method might suffice. For larger sets, hash tables or merge-based algorithms (for sorted sets) are significantly more efficient.
-
Data Structure: If the sets are already sorted arrays, the merge-based method is highly efficient. If using hash tables is feasible, they provide excellent performance.
-
Memory Constraints: Hash tables can consume more memory than other methods.
-
Availability of Built-in Functions: Database systems and programming languages often provide optimized set operations, which are generally the preferred approach if available.
Conclusion
The intersection operation is a cornerstone of set theory and has widespread applications in numerous domains. Understanding the different methods for implementing intersection, along with their respective time complexities and suitability for various data structures and scenarios, is vital for developers and anyone working with collections of data. By carefully considering the factors discussed above, one can choose the most efficient and appropriate method to perform set intersection, optimizing performance and resource utilization. The choice often involves a trade-off between time complexity and memory usage, with the optimal solution depending heavily on the specific application and constraints.
Latest Posts
Related Post
Thank you for visiting our website which covers about Which Of The Following Is A Method Of Intersection . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.