Algorithm For Converting Decimal To Binary
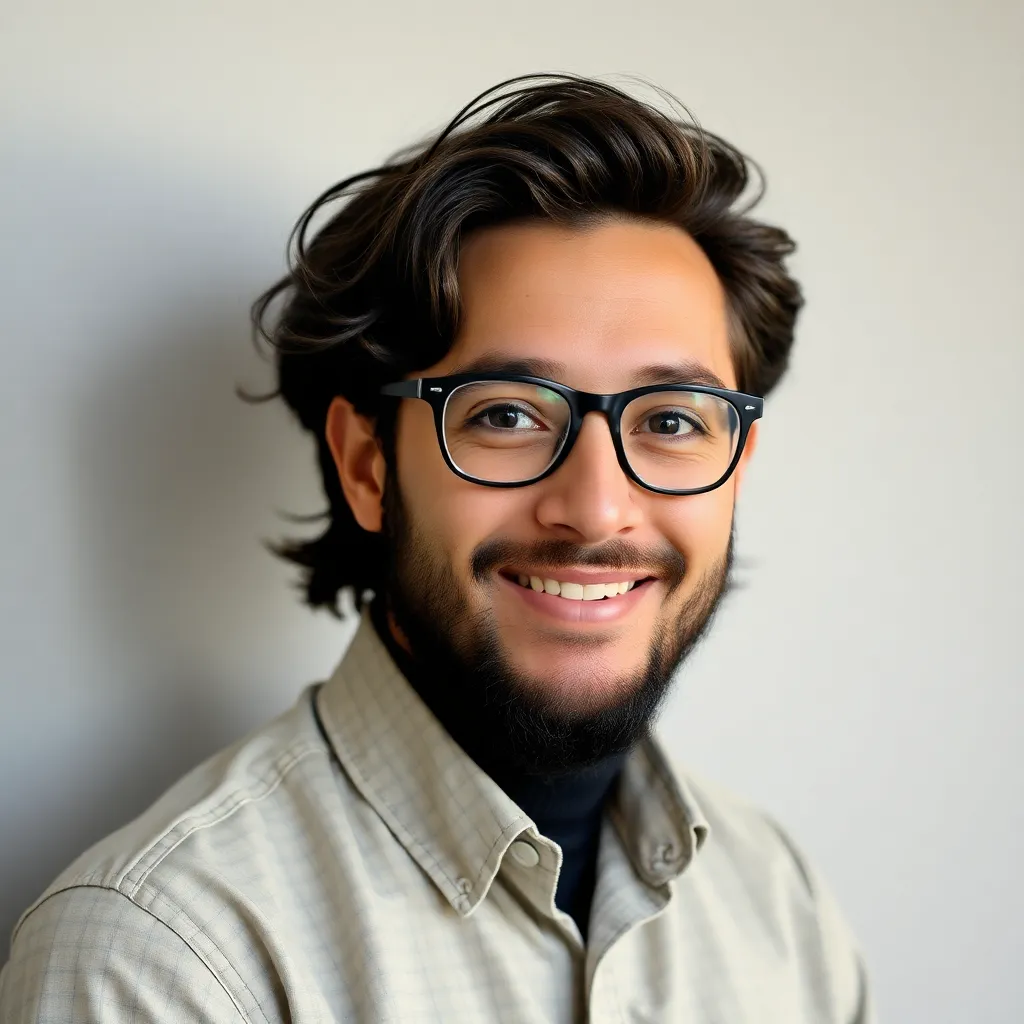
News Co
May 08, 2025 · 6 min read
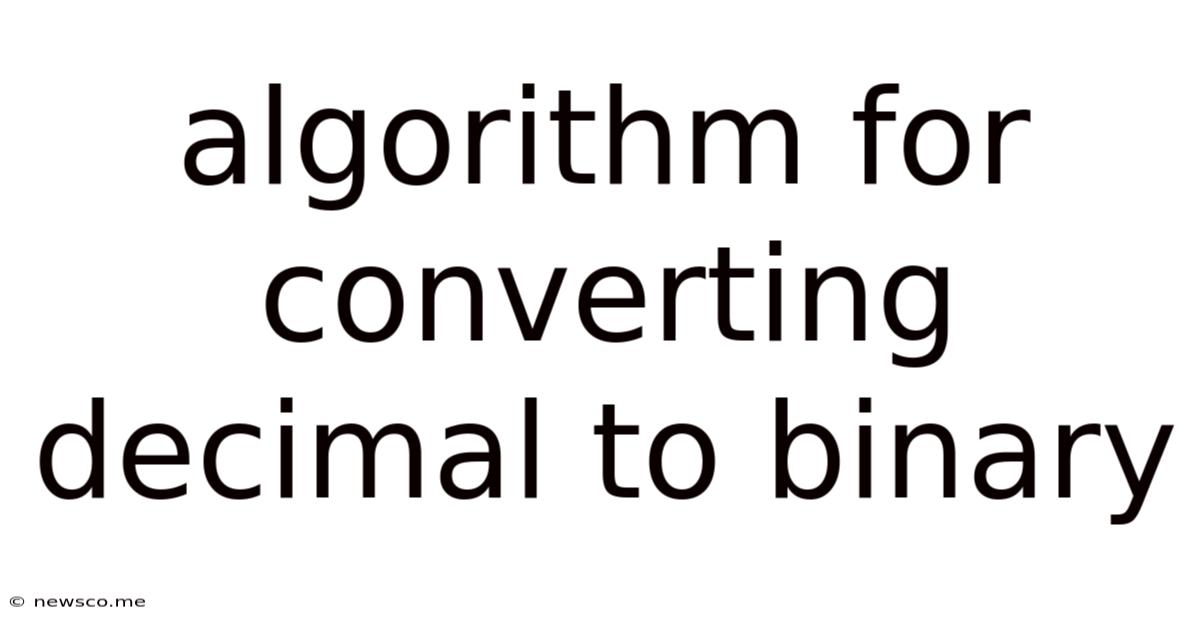
Table of Contents
Algorithms for Converting Decimal to Binary: A Deep Dive
Converting decimal numbers to their binary equivalents is a fundamental concept in computer science and digital electronics. Understanding the underlying algorithms allows for a deeper appreciation of how computers represent and manipulate numerical data. This comprehensive guide explores various algorithms for this conversion, ranging from simple methods suitable for manual calculations to more sophisticated approaches optimized for computational efficiency. We'll examine their strengths, weaknesses, and practical applications.
Understanding the Fundamentals: Decimal and Binary Number Systems
Before diving into the algorithms, let's briefly review the basics of decimal and binary number systems.
-
Decimal (Base-10): The decimal system, which we use daily, employs ten digits (0-9) and represents numbers using powers of 10. For instance, the number 123 is interpreted as (1 × 10²) + (2 × 10¹) + (3 × 10⁰).
-
Binary (Base-2): The binary system uses only two digits, 0 and 1, and represents numbers using powers of 2. For example, the binary number 1011 is equivalent to (1 × 2³) + (0 × 2²) + (1 × 2¹) + (1 × 2⁰) = 8 + 0 + 2 + 1 = 11 in decimal.
Algorithm 1: Repeated Division by 2 (The Most Common Method)
This is arguably the most widely used and easily understood algorithm for decimal-to-binary conversion. It involves repeatedly dividing the decimal number by 2 and recording the remainders. The remainders, read in reverse order, form the binary representation.
Steps:
- Divide: Divide the decimal number by 2.
- Record Remainder: Note down the remainder (either 0 or 1).
- Repeat: Repeat steps 1 and 2 with the quotient obtained in the previous step until the quotient becomes 0.
- Reverse: The binary representation is the sequence of remainders read from bottom to top (last remainder to the first).
Example: Convert decimal 13 to binary.
Division | Quotient | Remainder |
---|---|---|
13 ÷ 2 | 6 | 1 |
6 ÷ 2 | 3 | 0 |
3 ÷ 2 | 1 | 1 |
1 ÷ 2 | 0 | 1 |
Reading the remainders from bottom to top, we get 1101. Therefore, the binary representation of decimal 13 is 1101.
Python Implementation:
def decimal_to_binary(decimal_num):
"""Converts a decimal number to its binary equivalent."""
if decimal_num == 0:
return "0" #Handle the special case of 0
binary_representation = ""
while decimal_num > 0:
remainder = decimal_num % 2
binary_representation = str(remainder) + binary_representation #Prepend the remainder
decimal_num //= 2
return binary_representation
#Example usage
decimal_number = 25
binary_equivalent = decimal_to_binary(decimal_number)
print(f"The binary representation of {decimal_number} is: {binary_equivalent}")
Advantages: Simple, easy to understand and implement, works for all positive integers.
Disadvantages: Not the most efficient method for very large numbers, requires iterative process.
Algorithm 2: Using the Subtraction Method
This algorithm is a less common but conceptually interesting alternative. It iteratively subtracts the largest possible power of 2 from the decimal number until the result is 0.
Steps:
- Find Largest Power: Identify the largest power of 2 that is less than or equal to the decimal number.
- Subtract: Subtract this power of 2 from the decimal number.
- Record Bit: Record a '1' in the binary representation corresponding to the position of the power of 2 that was subtracted.
- Repeat: Repeat steps 1-3 with the result from step 2 until the result is 0. If a power of 2 cannot be subtracted, record a '0' in the corresponding position.
Example: Convert decimal 13 to binary.
- Largest power of 2 less than or equal to 13 is 8 (2³). Subtract 8, result is 5. Binary representation so far: 1???
- Largest power of 2 less than or equal to 5 is 4 (2²). Subtract 4, result is 1. Binary representation so far: 11??
- Largest power of 2 less than or equal to 1 is 1 (2⁰). Subtract 1, result is 0. Binary representation so far: 1101
- Result is 0, the process is complete. The binary representation is 1101.
Python Implementation:
def decimal_to_binary_subtraction(decimal_num):
"""Converts a decimal number to binary using subtraction."""
if decimal_num == 0:
return "0"
binary_representation = ""
power_of_2 = 1
while decimal_num > 0:
if decimal_num >= power_of_2:
binary_representation = "1" + binary_representation
decimal_num -= power_of_2
else:
binary_representation = "0" + binary_representation
power_of_2 *= 2
return binary_representation
#Example Usage:
decimal_number = 25
binary_equivalent = decimal_to_binary_subtraction(decimal_number)
print(f"The binary representation of {decimal_number} is {binary_equivalent}")
Advantages: Provides a different perspective on the conversion process.
Disadvantages: Less efficient than repeated division, especially for larger numbers. Requires careful tracking of powers of 2.
Algorithm 3: Using Bitwise Operators (For Advanced Users)
For those familiar with bitwise operations, a highly efficient approach leverages the bitwise AND operator (&). This method directly manipulates bits, making it exceptionally fast for computers.
Steps:
- Iterate: Iterate through each bit position, starting from the least significant bit.
- Bitwise AND: Perform a bitwise AND operation between the decimal number and 1. The result will be either 0 or 1, representing the least significant bit.
- Right Shift: Right-shift the decimal number by 1 bit using the bitwise right shift operator (>>). This effectively moves the next bit into the least significant position.
- Repeat: Repeat steps 2 and 3 until all bits have been processed.
Example (Conceptual): The detailed explanation with bit manipulation would be too extensive for this text format, but the core concept is to leverage bitwise operations for speed and efficiency.
Python Implementation:
def decimal_to_binary_bitwise(decimal_num):
"""Converts a decimal number to binary using bitwise operators."""
if decimal_num == 0:
return "0"
binary = ""
while decimal_num > 0:
binary = str(decimal_num & 1) + binary
decimal_num >>= 1
return binary
#Example Usage:
decimal_number = 25
binary_equivalent = decimal_to_binary_bitwise(decimal_number)
print(f"The binary representation of {decimal_number} is: {binary_equivalent}")
Advantages: Extremely efficient, directly manipulates bits at the hardware level.
Disadvantages: Requires understanding of bitwise operations; less intuitive for beginners.
Algorithm 4: Using Built-in Functions (Python Specific)
Python offers built-in functions to simplify the conversion process. The bin()
function directly converts a decimal integer into its binary string representation.
Example:
decimal_number = 13
binary_equivalent = bin(decimal_number)
print(f"The binary representation of {decimal_number} is: {binary_equivalent}") # Output includes "0b" prefix.
# Removing the "0b" prefix:
print(f"The binary representation of {decimal_number} is: {binary_equivalent[2:]}")
Advantages: Extremely concise and easy to use.
Disadvantages: Relies on a language-specific feature, not a general algorithmic approach suitable for all programming languages.
Choosing the Right Algorithm
The best algorithm for decimal-to-binary conversion depends on the context and priorities.
- For educational purposes and manual calculations: The repeated division by 2 method is the clearest and easiest to understand.
- For efficient implementation in code: The bitwise operator method offers the highest performance, particularly for large numbers.
- For rapid prototyping or scripting: The built-in function (e.g.,
bin()
in Python) provides the quickest solution.
This comprehensive exploration of decimal-to-binary conversion algorithms equips you with the knowledge and tools to choose the most appropriate approach based on your specific needs. Understanding these algorithms not only enhances your comprehension of number systems but also lays a solid foundation for more advanced topics in computer science and digital systems design. Remember to choose the algorithm that best balances efficiency, readability, and ease of implementation for your project.
Latest Posts
Latest Posts
-
How Much Is 270 Pounds In Us Dollars
May 08, 2025
-
Which Fraction Is Equivalent To 4 5
May 08, 2025
-
413 Divided By 2 With Remainder
May 08, 2025
-
Which Side Of A Rectangle Is The Length
May 08, 2025
-
How Many Quarts In A Litre
May 08, 2025
Related Post
Thank you for visiting our website which covers about Algorithm For Converting Decimal To Binary . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.